All About Network Sockets
What is as Socket?
A socket is one endpoint of a two-way communication link between two programs running on the network.
Types of Sockets
Stream Socket
- Also called connection-oriented sockets
- Sends and receives messages through streams (much like reading/writing data from files)
- Doesn't care about message boundaries
- Commonly used for TCP
Datagram Socket
- Also called connectionless sockets
- Sends and receives messages as independent packets with defined boundaries (i.e. one receive = one message)
- Commonly used for UDP
Raw Socket
- Allows direct sending and receiving of IP packets without any protocol-specific transport layer formatting (basically skips the transport layer)
- Has no concept of ports
- Can be used if you want to implement your own transport protocol
- Also used by network utility programs such as nmap, ping, etc.
Socket API
A collection of functions/methods/commands usually provided by the operating system to enable programs to use sockets and communicate with other programs.
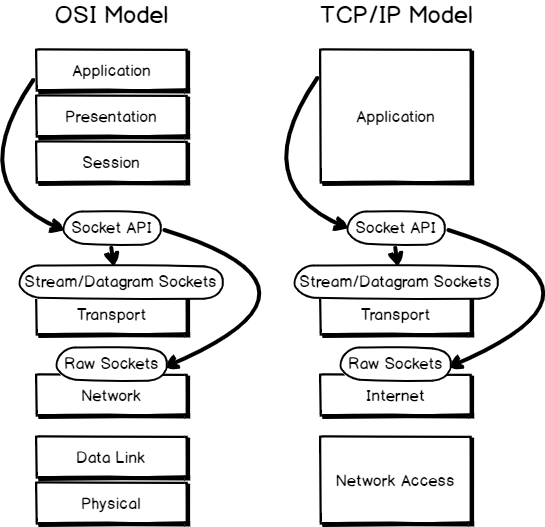
Berkeley Sockets
- Also called connectionless sockets
- Sends and receives messages as independent packets with defined boundaries (i.e. one receive = one message)
- Commonly used for UDP
List of Common Berkeley Socket API Functions
socket()
Instantiates a socket object
bind()
Binds an IP address and port number to a socket object
connect()
Establishes a connection to a remote host
listen()
Used by servers to listen for incoming connections
accept()
Accepts a connection request from the listen() function and returns a new socket associated with the new connection
send()
Sends data through the socket
recv()
Receives data from a socket
close()
Closes the socket
Other common socket functions include:
- read(), recvfrom(), or recvmsg()
- write(), sendto(), or sendmsg()
- gethostbyname() and gethostbyaddr()
TCP Socket API Call Sequence
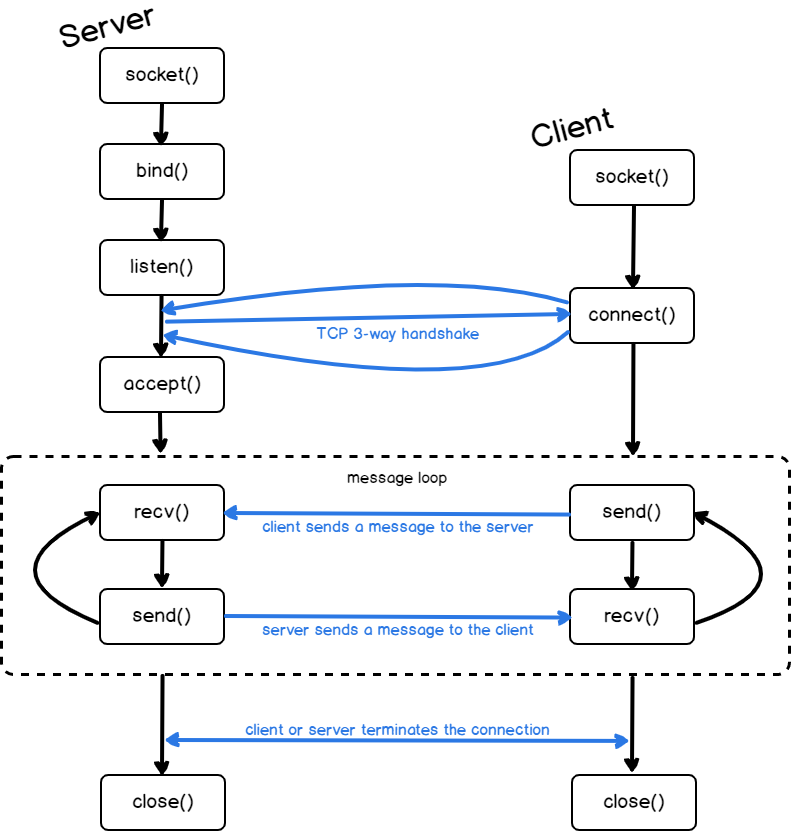
UDP Socket API Call Sequence
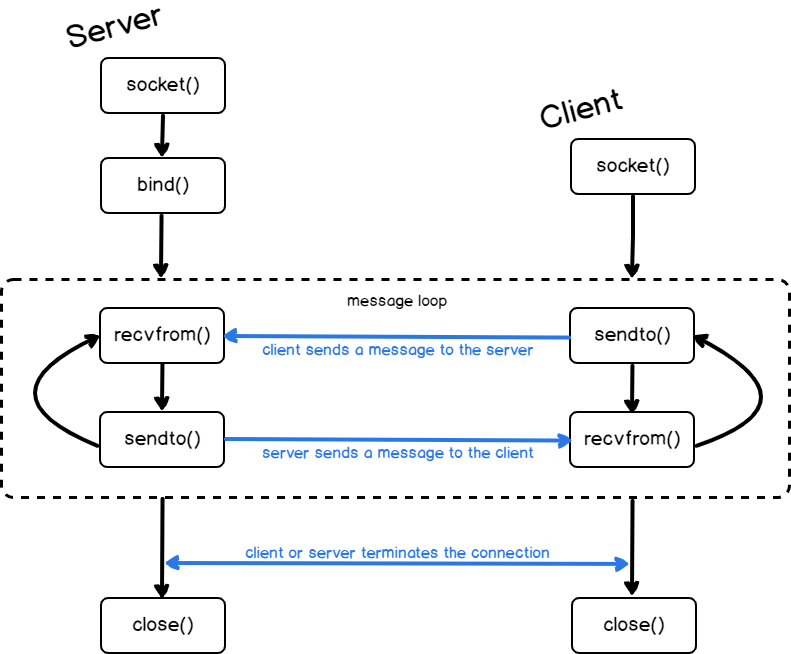
Socket Protocols
- IPPROTO_TCP for TCP
- IPPROTO_UDP for UDP
- Other values include IPPROTO_SCTP and IPPROTO_DCCP
Note that you can use the same port number for different protocols.
Socket Domains (aka Protocol Family/Address Family)
AF_INET/PF_INET
- Corresponds to IPv4
- AddressFamily.InterNetwork in the .NET implementation
AF_INET6/PF_INET6
- Corresponds to IPv6
- AddressFamily.InterNetworkV6 in the .NET implementation
AF_UNIX/PF_UNIX
- Corresponds to local sockets (using a file)
- Used in UNIX for inter-process communication (IPC)
Socket Operating Modes
Blocking Mode
- When operating in Blocking mode, some socket functions such as accept(), send(), and recv() will block execution until the operation is successful. The accept() function for instance, will wait until a client initiates a connection to the server.
- In .NET, this can be set by assigning true to the Socket.Blocking property. This is the default value in .NET.
Non-Blocking Mode
- In non-blocking mode, socket functions will not block execution.
- In .NET, this can be set by assigning false to the Socket.Blocking property.
Sources:
https://en.wikipedia.org/wiki/Network_socket
https://en.wikipedia.org/wiki/Berkeley_sockets
https://docs.oracle.com/javase/tutorial/networking/sockets/definition.html
http://homepage.smc.edu/morgan_david/cs70/sockets.htm
http://ijcsit.com/docs/Volume%205/vol5issue03/ijcsit20140503462.pdf
https://docs.microsoft.com/en-us/dotnet/framework/network-programming